C++静态成员变量详解
默认情况下,每个类的对象(类的一个实例)都有它自己的类的成员变量副本。一个对象的成员变量与同一个类的其他对象的成员变量是独立分开并且有区别的。
例如,来看以下声明:
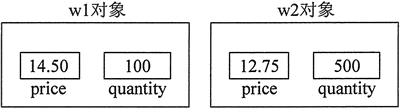
图 1 两个不同的对象 w1 和 w2
当任意一个实例的 getQuantity 成员函数被调用时,它将返回存储在调用对象的 quantity 变量中的值。基于最初存储在各自对象中的值,以下声明将显示 2 个不同的结果:
可以创建一个由同一个类的所有对象共享的成员变量。要创建这样的成员,只需将关键字 static 放在变量声明的前面,如下面的类所示:
例如,假设程序中出现以下语句:
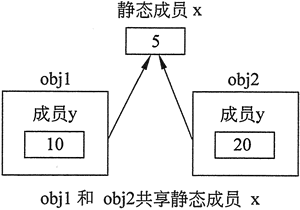
图 2
下面的程序演示了一个更实用的静态成员变量用法。
Budget 类用于收集公司所有部门的预算请求。该类使用静态成员 corpBudget 来保存整个公司预算的金额。当成员函数 addBudget 被调用时,它的实参被添加到 corpBudget 的当前内容中。在程序完成时,corpBudget 将包含所有 Budget 类对象放置的所有值的总和。
一般来说,可以将一个类的成员变量分成两组:实例成员和静态成员。实例成员是其使用必须与类的特定实例关联的成员。特别是,一个类的实例变量必须通过该类的特定实例来访问。
相比之下,静态成员变量的使用就不需要与任何实例关联。只有静态成员的类需要指定。
例如,来看以下声明:
class Widget { private: double price; int quantity; public: Widget(double p, int q) { price = p; quantity = q; } double getPrice() const { return price; } int getQuantity() const { return quantity; } };假定在某个程序中,使用以下声明创建了 Widget 类的两个单独的实例:
Widget w1(14.50, 100), w2(12.75, 500);
该语句创建了两个不同的对象 w1 和 w2。每个对象都有自己的 price 和 quantity 成员变量,如图 1 所示。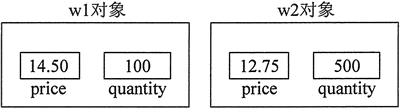
图 1 两个不同的对象 w1 和 w2
当任意一个实例的 getQuantity 成员函数被调用时,它将返回存储在调用对象的 quantity 变量中的值。基于最初存储在各自对象中的值,以下声明将显示 2 个不同的结果:
cout << w1.getQuantity () << " " << w2.getQuantity();
以上代码的显示结果为 "100 500"。可以创建一个由同一个类的所有对象共享的成员变量。要创建这样的成员,只需将关键字 static 放在变量声明的前面,如下面的类所示:
class StatDemo { private: static int x; int y; public: void setx(int a) const { x = a; } void sety(int b) const { y = b; } int getx() { return x; } int gety() { return y; } };接下来,在类之外放置一个单独的变量定义,例如:
int StatDemo::x;
在这个例子中,成员变量 x 将被 StatDemo 类的所有对象共享。当一个类对象将一个值放入 x 中时,它将出现在所有其他 StatDemo 对象中。例如,假设程序中出现以下语句:
StatDemo obj1, obj 2; obj1.setx(5); obj1.sety(10); obj2.sety(20); cout << "x: " << obj1.getx () << " " << obj2.getx() << endl; cout << "y: " << obj1.gety () << " " << obj2.gety() << endl;cout 语句将显示以下内容:
x: 5 5
y: 10 20
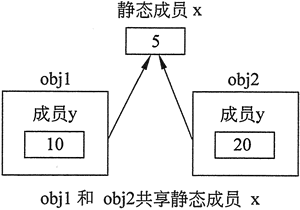
图 2
下面的程序演示了一个更实用的静态成员变量用法。
Budget 类用于收集公司所有部门的预算请求。该类使用静态成员 corpBudget 来保存整个公司预算的金额。当成员函数 addBudget 被调用时,它的实参被添加到 corpBudget 的当前内容中。在程序完成时,corpBudget 将包含所有 Budget 类对象放置的所有值的总和。
//budget.h的内容 #ifndef BUDGET_H #define BUDGET_H class Budget { private: static double corpBudget; double divBudget; public: Budget() {divBudget =0;} void addBudget(double b) {divBudget += b; corpBudget += divBudget; } double getDivBudget() const { return divBudget; } double getCorpBudget() const { return corpBudget; } }; #endif //main程序的内容 // This program demonstrates a static class member variable. #include <iostream> #include <iomanip> #include "budget.h" // For Budget class declaration using namespace std; // Definition of the static member of the Budget class double Budget::corpBudget = 0; int main() { const int N_DIVISIONS = 4; Budget divisions[N_DIVISIONS]; // Get the budget request for each division for (int count = 0; count <N_DIVISIONS; count++) { double bud; cout << "Enter the budget request for division "; cout << (count + 1) << ": "; cin >> bud; divisions[count].addBudget(bud); } // Display the budget request for each division cout << setprecision(2); cout << showpoint << fixed; cout << "\nHere are the division budget requests: \n"; for (int count =0; count < N_DIVISIONS; count++) { cout << "Division " << (count + 1) << "\t$ "; cout << divisions[count].getDivBudget() << endl; } // Display the total budget request cout << "Total Budget Requests:\t$ "; cout << divisions[0].getCorpBudget() << endl; return 0; }程序输出结果:
Enter the budget request for division 1:102000
Enter the budget request for division 2:202000
Enter the budget request for division 1:570000
Enter the budget request for division 1:100100
Here are the division budget requests:
Division 1 $ 102000.00
Division 2 $ 201000.00
Division 3 $ 570000.00
Division 4 $ 100100.00
Total Budget Requests: $ 973100.00
一般来说,可以将一个类的成员变量分成两组:实例成员和静态成员。实例成员是其使用必须与类的特定实例关联的成员。特别是,一个类的实例变量必须通过该类的特定实例来访问。
相比之下,静态成员变量的使用就不需要与任何实例关联。只有静态成员的类需要指定。
所有教程
- C语言入门
- C语言编译器
- C语言项目案例
- 数据结构
- C++
- STL
- C++11
- socket
- GCC
- GDB
- Makefile
- OpenCV
- Qt教程
- Unity 3D
- UE4
- 游戏引擎
- Python
- Python并发编程
- TensorFlow
- Django
- NumPy
- Linux
- Shell
- Java教程
- 设计模式
- Java Swing
- Servlet
- JSP教程
- Struts2
- Maven
- Spring
- Spring MVC
- Spring Boot
- Spring Cloud
- Hibernate
- Mybatis
- MySQL教程
- MySQL函数
- NoSQL
- Redis
- MongoDB
- HBase
- Go语言
- C#
- MATLAB
- JavaScript
- Bootstrap
- HTML
- CSS教程
- PHP
- 汇编语言
- TCP/IP
- vi命令
- Android教程
- 区块链
- Docker
- 大数据
- 云计算