C语言求定积分
利用梯形法计算定积分
其中, f(x)=x3+3x2-x+2。
若右边的极限存在,其极限值即为定积分的值。理论上区间分得越细,越逼近定积分实际的值,一般采用梯形法近似计算定积分的值,把区间 [a,6] 划分成 n 等份,则任意第 f 个小梯形的面积为 (上底+下底)×高/2,si=H×[f(xi)-1)+f(xi)]/2,其中 xi+1=a+(i+1)×H;xi=a+i×H;H=(b-a)/n。该实例问题实际上转换为求 n 等份梯形的面积累计和。
② 函数也是有地址的,函数名作为函数的首地址。可以定义一个指向函数的指针变量,将函数入口地址赋予指针变量,然后通过指针变量调用函数,这样的指针变量即称为指向函数的指针。
③ 函数指针也是指针变量,可以实现指针变量的运算,但不能进行算术运算,因为函数指针的移动是毫无意义的,不同于数组指针变量,加减一个整数可以使指针指向后面或前面的数组元素。
④ 在函数调用中 “(* 指针变量名)” 两边的括号不可少,其中此处为一种表示符号,而不是求值运算。
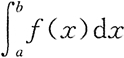
算法思想
根据定积分的定义分析可得:[x0,x1],[x1,x2],···,[xn-1,xn],将定积分的区间 [a,b] 分成 n 个子区间,其中:
若右边的极限存在,其极限值即为定积分的值。理论上区间分得越细,越逼近定积分实际的值,一般采用梯形法近似计算定积分的值,把区间 [a,6] 划分成 n 等份,则任意第 f 个小梯形的面积为 (上底+下底)×高/2,si=H×[f(xi)-1)+f(xi)]/2,其中 xi+1=a+(i+1)×H;xi=a+i×H;H=(b-a)/n。该实例问题实际上转换为求 n 等份梯形的面积累计和。
程序代码
#include <stdio.h> #include <math.h> float collect(float s,float t,int m,float (*p)(float x)); float fun1(float x); float fun2(float x); float fun3(float x); float fun4(float x); int main() { int n,flag; float a,b,v=0.0; printf("Input the count range(from A to B)and the number of sections.\n"); scanf("%f%f%d",&a,&b,&n); printf("Enter your choice:'1' for fun1,'2' for fun2,'3' for fun3,'4' for fun4==>"); scanf("%d",&flag); if(flag==1) v=collect(a,b,n,fun1); else if(flag==2) v=collect(a,b,n,fun2); else if(flag==3) v=collect(a,b,n,fun3); else v=collect(a,b,n,fun4); printf("v=%f\n",v); return 0; } float collect(float s,float t,int n,float (*p)(float x)) { int i; float f,h,x,y1,y2,area; f=0.0; h=(t-s)/n; x=s; y1=(*p)(x); for(i=1;i<=n;i++) { x=x+h; y2=(*p)(x); area=(y1+y2)*h/2; y1=y2; f=f+area; } return (f); } float fun1(float x) { float fx; fx=x*x-2.0*x+2.0; return(fx); } float fun2(float x) { float fx; fx=x*x*x+3.0*x*x-x+2.0; return(fx); } float fun3 (float x) { float fx; fx=x*sqrt(1+cos(2*x)); return(fx); } float fun4(float x) { float fx; fx=1/(1.0+x*x); return(fx); }
调试运行结果
程序运行结果如下所示:
Input the count range(from A to B)and the number of sections.
0 1 100
Enter your choice:'1' for fun1,'2' for fun2,'3' for fun3,'4' for fun4==>2
v=2.750073
总结
① 定义 collect() 函数时,函数的首部 “float collect(float s,float t,int n,float (*p)(float x))” 中的 “float (*p)(float x)” 表示 p 是指向函数的指针变量,该函数的形参为实型。在 main() 函数的 if 条件结构中调用 collect() 函数时,除了将 a,b,n 作为实参传给 collect 的形参 s,n,t 外,还必须将函数名 fun1,fun2,fun3,fun4 作为实参将其入口地址传递给 collect() 函数中的形参 p。② 函数也是有地址的,函数名作为函数的首地址。可以定义一个指向函数的指针变量,将函数入口地址赋予指针变量,然后通过指针变量调用函数,这样的指针变量即称为指向函数的指针。
③ 函数指针也是指针变量,可以实现指针变量的运算,但不能进行算术运算,因为函数指针的移动是毫无意义的,不同于数组指针变量,加减一个整数可以使指针指向后面或前面的数组元素。
④ 在函数调用中 “(* 指针变量名)” 两边的括号不可少,其中此处为一种表示符号,而不是求值运算。
所有教程
- C语言入门
- C语言编译器
- C语言项目案例
- 数据结构
- C++
- STL
- C++11
- socket
- GCC
- GDB
- Makefile
- OpenCV
- Qt教程
- Unity 3D
- UE4
- 游戏引擎
- Python
- Python并发编程
- TensorFlow
- Django
- NumPy
- Linux
- Shell
- Java教程
- 设计模式
- Java Swing
- Servlet
- JSP教程
- Struts2
- Maven
- Spring
- Spring MVC
- Spring Boot
- Spring Cloud
- Hibernate
- Mybatis
- MySQL教程
- MySQL函数
- NoSQL
- Redis
- MongoDB
- HBase
- Go语言
- C#
- MATLAB
- JavaScript
- Bootstrap
- HTML
- CSS教程
- PHP
- 汇编语言
- TCP/IP
- vi命令
- Android教程
- 区块链
- Docker
- 大数据
- 云计算